Tetris.JS
Recreating my favorite puzzle game for the web
This project is a Tetris game built using React, TypeScript, and Vite. It features a fully functional Tetris game with modern web development practices, including hot module replacement (HMR). The game includes various features such as piece statistics, score tracking, audio controls, and more.
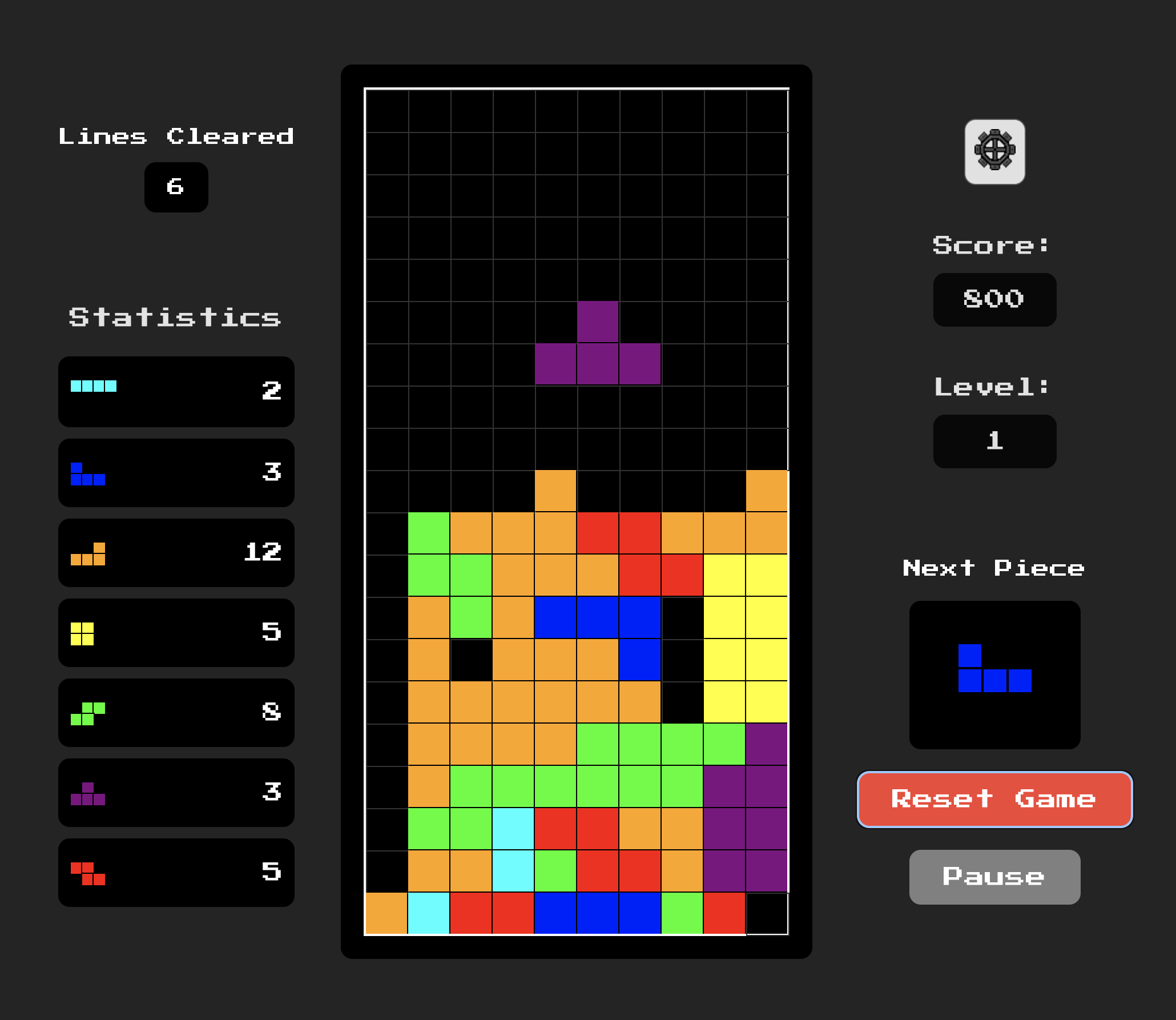
Key Features
- Game control functions using the arrow keys
- Audio and SFX controls
- Score tracking
- Piece statistics
- Game over screen
- Start menu
- Settings menu
- Hot module replacement (HMR)
- ESLint for code quality
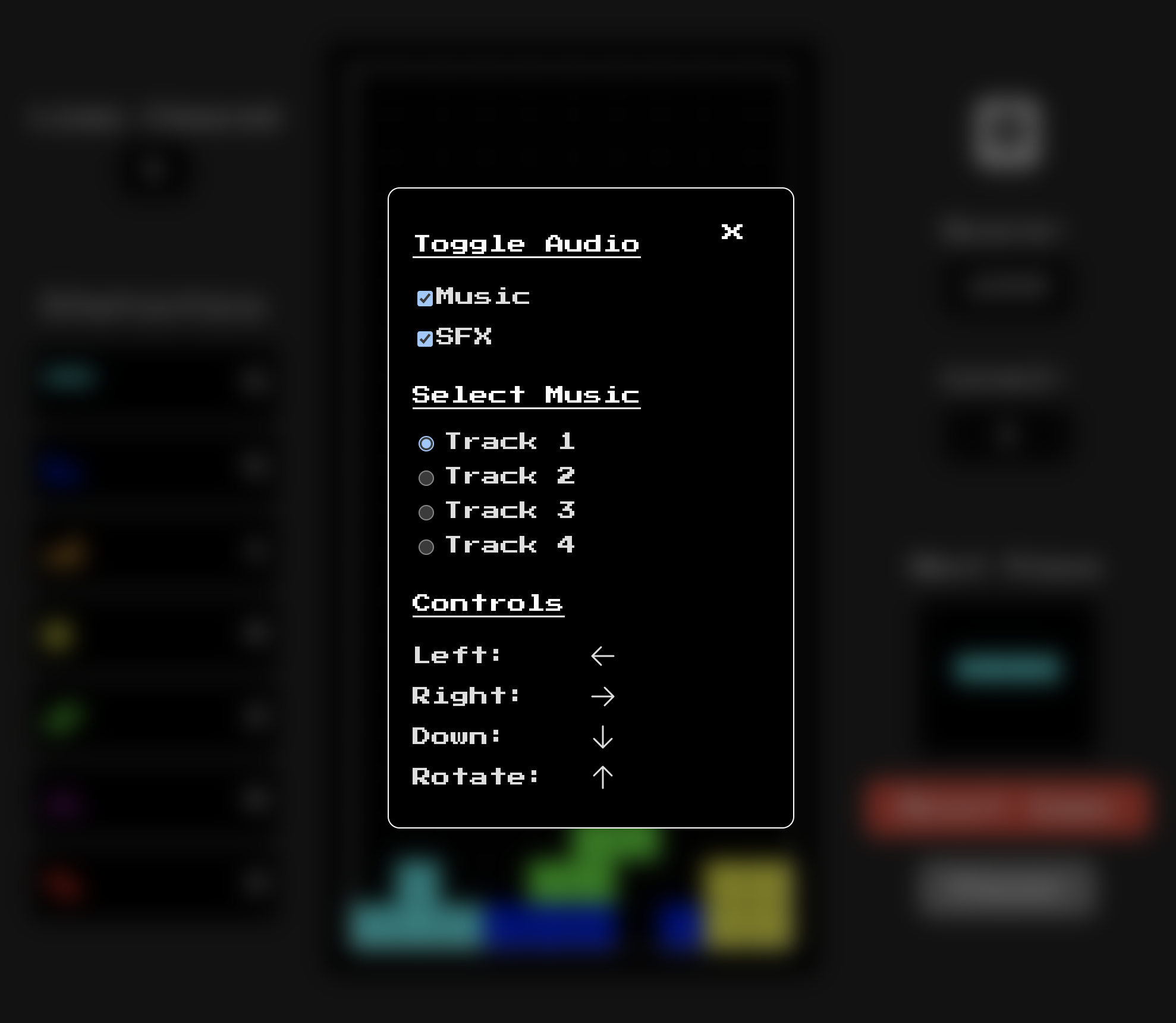
Technologies Used
- React
- TypeScript
- Vite
- ESLint
- TailwindCSS
Implementation Details
The game logic for this Tetris game is implemented using a custom React hook called useGameLogic
. This hook manages the state and behavior of the game, including piece movements, collision detection, line clearing, scoring, and level progression. Below is a summary of the key components and functions within the game logic:
Random Tetromino Bag
The initial implementation of the random tetromino generator was completely random, which meant that the same tetromino would often appear in a row and caused scenarios where if felt like the game was not random. After doing some reseach I discovered versions of the tetris game used a bag-7 randomizer which randomizes the tetromino bag after every 7 tetrominos. This still kept the game random without the scenarios where there was a drought of a particular piece. This implementation uses the Fisher-Yates shuffle algorithm to randomize the tetromino bag.
Here is the code for the Bag-7, Fisher-Yates shuffle algorithm:
const shuffleArray = (array: (keyof typeof TETROMINOS)[]): (keyof typeof TETROMINOS)[] => {
const shuffled = [...array];
for (let i = shuffled.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1));
[shuffled[i], shuffled[j]] = [shuffled[j], shuffled[i]];
}
return shuffled;
};
See the before and after of the bag-7 randomization below:
Before Bag-7 Randomization:
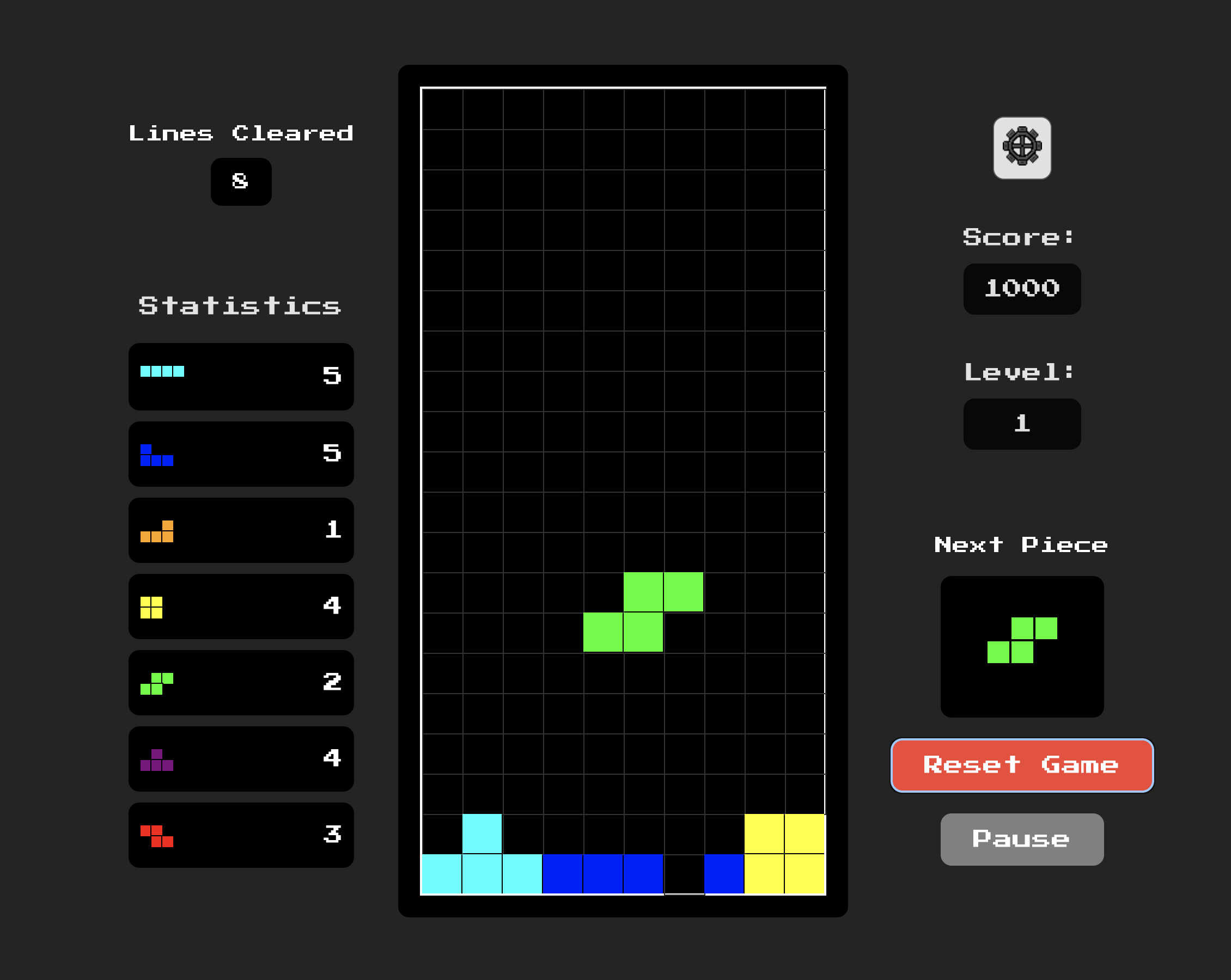
After Bag-7 Randomization:
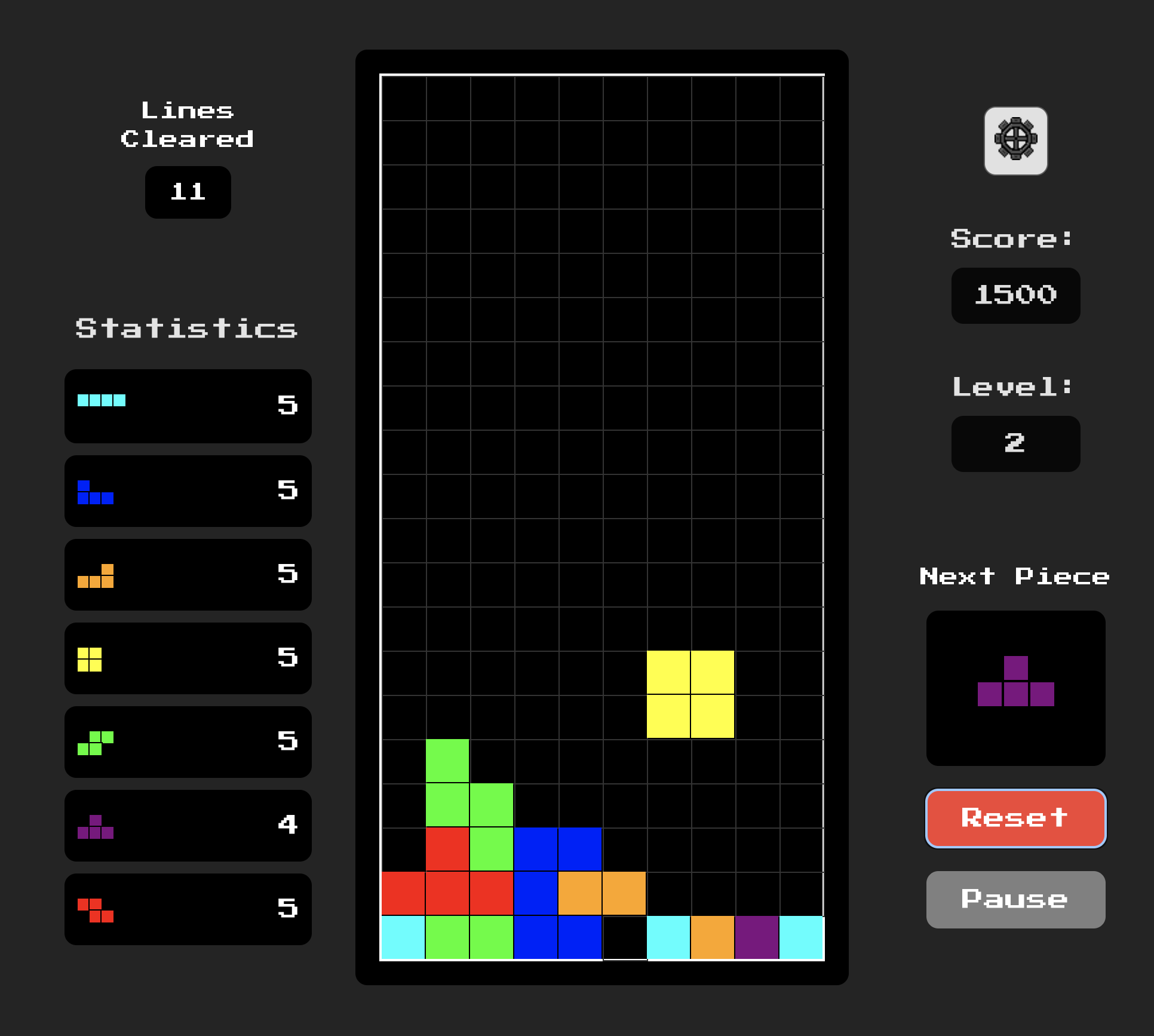
Next Steps
- Add a leaderboard
- Include other game modes such as puzzle, endless, and co-op
- Refine game aesthetics
- Update movement and rotation logic to be more efficient and more similar to the NES tetris game
- Mobile support